The Software Architecture Handbook
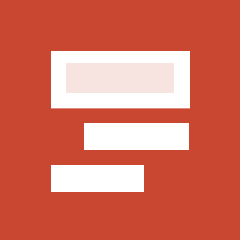
- Author: freecodecamp.org
- Full Title: The Software Architecture Handbook
- Type: #snippet✂️
- URL: https://www.freecodecamp.org/news/an-introduction-to-software-architecture-patterns/
Highlights
- The simplest way I can put it is, software architecture refers to how you organize stuff in the process of creating software. And "stuff" here can refer to: Implementation details (that is, the folder structure of your repo) Implementation design decisions (Do you use server side or client side rendering? Relational or non-relational databases?) The technologies you choose (Do you use REST or GraphQl for your API? Python with Django or Node with Express for your back end?) System design decisions (like is your system a monolith or is it divided into microservices?) Infrastructure decisions (Do you host your software on premise or on a cloud provider?)
- Client-server is a model that structures the tasks or workloads of an application between a resource or service provider (server) and a service or resource requester (client). Put simply, the client is the application that requests some kind of information or performs actions, and the server is the program that sends information or performs actions according to what the client does. Clients are normally represented by front-end applications that run either on the web or mobile apps (although other platforms exist too and back-end applications can act as clients as well). Servers are usually back-end applications.
- An API is nothing more than a set of defined rules that establishes how an application can communicate with another. It's like a contract between the two parts that says "If you send A, I'll always respond B. If you send C, I'll always respond D..." and so on. Having this set of rules, the client knows exactly what it has to require in order to complete a certain task, and the server knows exactly what the client will require when a certain action has to be performed. There're different ways in which an API can be implemented. The most commonly used are REST, SOAP and GraphQl.
- So if you're new to all this you might be thinking "what on earth is a microservice", right? Well, we could define it as the concept of dividing server side features into many small servers that are responsible for only one or a few specific features.
- What is back-end for front-end (BFF)? One problem that comes up when implementing microservices is that the communication with front-end apps gets more complex. Now we have many servers responsible for different things, which means front-end apps would need to keep track of that info to know who to make requests to. Normally this problem gets solved by implementing an intermediary layer between the front-end apps and the microservices. This layer will receive all the front-end requests, redirect them to the corresponding microservice, receive the microservice response, and then redirect the response to the corresponding front-end app.
- Before we mentioned that vertically scaling means adding more resources (RAM, disk space, GPU, and so on) to a single server/computer. Horizontally scaling on the other hand, means setting up more servers to perform the same task.
- This distribution of requests is normally performed by a thing called a load balancer. Load balancers act as reverse proxys to our servers, intercepting client requests before they get to the server and redirecting that request to the corresponding server.
- Traditional The first way is to use them in a similar way you'd use a traditional server provider. You select the kind of hardware you want and pay exactly for that on a monthly basis.
- Elastic The second way is to take advantage of the "elastic" computing offered by most providers. "Elastic" means that the hardware capacity of your application will automatically grow or shrink depending on the usage your app has.
- Serverless Another way in which you can use cloud computing is with a serverless architecture. Following this pattern, you wont have a server that receives all requests and responds to them. Instead you'll have individual functions mapped to an access point (similar to an API endpoint). These functions will execute each time they receive a request and perform whatever action you programmed them for (connecting to a database, performing CRUD operations or whatever else a you could do in a regular server).
- The application layer will have the basic setup of our server and the connection to our routes (the next layer). The routes layer will have the definition of all of our routes and the connection to the controllers (the next layer). The controllers layer will have the actual logic we want to perform in each of our endpoints and the connection to the model layer (the next layer, you get the idea...) The model layer will hold the logic for interacting with our mock database. Finally, the persistence layer is where our database will be.
- MVC is an architecture pattern that stands for Model View Controller. We could say the MVC architecture is like a simplification of the layers architecture, incorporating the front-end side (UI) of the application as well. Under this architecture, we'll have only three main layers: The view layer will be responsible for rendering the UI. The controllers layer will be responsible for defining routes and the logic for each of them. The model layer will be responsible for interacting with our database.